Welcome to part 4 in our series of blogposts where we introduce beginners to programming.
Be sure to read the following previous episodes:
Introduction
Arrays and lists are what they sound like. Whether you use the term array or list depends on what language you’re in. A lot of languages use arrays while Python uses lists which is basically similar to arrays (C++ has something else also called lists). For the rest of this post, except in Python-specific contexts, we’ll call them arrays.
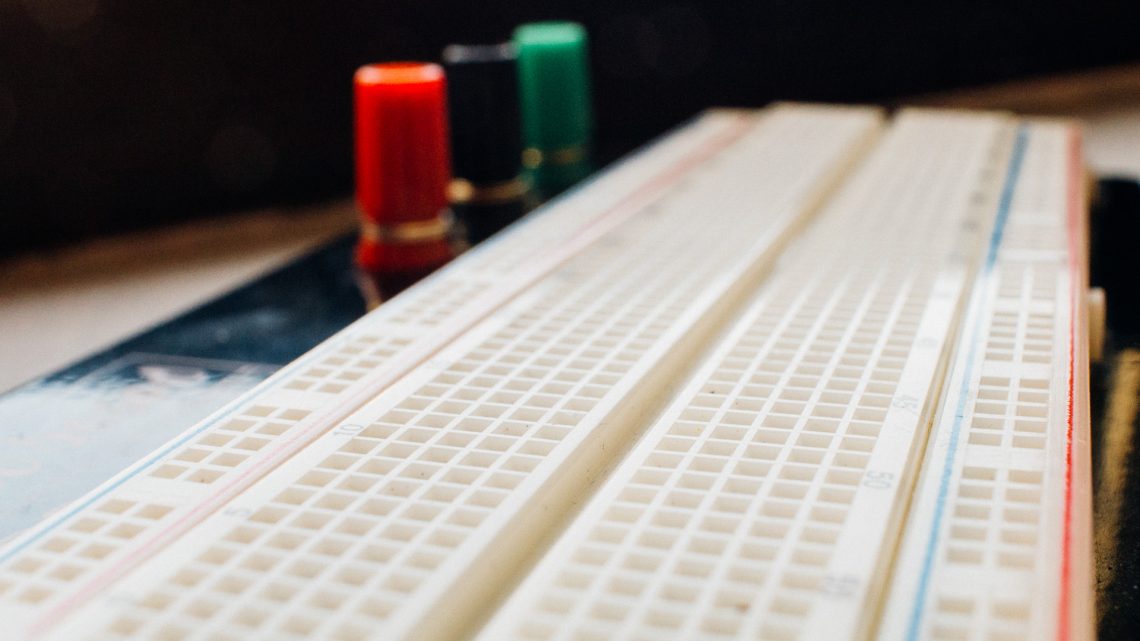
An array is basically a chain (or list) of elements. An element in this context can be a simple variable or more complex data types such as structs or objects (we’ll focus on simple variables for now). Every element in the array has its own index which decides where in the chain the variable is located.
Zero-indexation
Arrays are more often than not zero-indexed which means that the first variable in the array has index 0, the second has index 1, the third index 2 and so on. We actually call the first variable the zeroth element and the second variable the first element to keep things compatible with the zero-indexation.
Dimensions
Arrays can have many dimensions. The standard array has one dimension. A two-dimensional array forms a typical table or matrix as you see in spreadsheets and such and is basically many arrays within a single array. A three-dimensional array forms a 3D body like a Rubik’s Cube. You can also have more dimensions which quickly falls into the imaginary domain.
Why Do We Use Arrays?
There are no limits to what arrays can be used for. It’s mainly a way to store and organize variables to later look them up and/or change them. Arrays can represent virtual or real life objects such as a computer screen (two dimensions) where every element is one pixel, or for instance a Minecraft-like world (three dimensions) where every element is a block.
Arrays are however more often than not used in much more abstract situations where you just need to organize a set of variables in some way.
In part 3 we used an example where we printed out the first n numbers in the Fibonacci sequence. Instead of just printing them out we could’ve stored them in an array so that we later could’ve looked up different numbers (we’ll do this in the examples towards the end).
Declaring Arrays
Arduino/C++ (and many other languages) differs quite a bit from Python when it comes arrays.
Arduino/C++
When doing this in Arduino/C++ you have to specify the length of the array (number of elements) when declaring it. This number must be constant! The compiler will then reserve a cunk of memory for that array. Then, depending on the platform the code is running on, if you’re trying to access element number 54 in a 10 element long array during while the program is running, one of two things will happen:
- On a computer with memory protection an error will occur to avoid doing things you shouldn’t do.
- On a microcontroller without memory protection element number 54 will be a totally random chunk of memory. Reading it will probably return a random value while writing to it might corrupt important registers so that the microcontroller stops working.
There are several alternatives to the standard type of arrays (e.g. vectors), but in this post we are just going to look at the basic type of arrays. In these languages (unlike Python) the elements in an array has to be of the same type!
Examples
Below is four ways to declare (and initialize) arrays.
int my_array[10]; int my_int_array[5] = {3, 2, 5, 7, 3}; string my_string_array[3] = {"hey", "hi", "hello"}; int my_zero_array[100] = { };
- The first array my_array is just declared and not initialized. This means that we don’t yet know what the values of the elements are.
- The second array my_int_array is explicitly initilized with the integers within the curly brackets.
- my_string_array is declared and initialized the same way as my_int_array, except that the array is of the type string. If we try to initalize the array with different types than the type of the array we will get a compilation error.
- my_zero_array is initialized with the value 0 at each element.
Python
Python is a bit more liberal when it comes to the size of lists. You can create a list with a certain length filled in with some value for every element (like in C++/Arduino), or you can create a list with zero elements and add elements later on (or a combination of the two). If you try to access an element which is outside of the range of the list (except when adding new elements to the list) you will get an error and the program will stop running. Elements in a list can be of different types.
You can have multiple types of variables within a single Python list.
Examples
Below is three ways to declare (and initialize) lists.
my_empty_list = [] my_initialized_list = [1, "cake", 6.43] my_zero_list = [0]*42
- The first list is completely empty. You are not able to access any of its elements because it doesn’t have any.
- my_initialized_list is three elements long and contains has multiple data types.
- The last list is fourty-two elements long list with the value 0 for each element.
Accessing Elements for Reading and Writing
This is a similar operation in both C++/Arduino and Python. Both of these languages use zero-indexation as well.
C++/Arduino
The following code sets the variable my_var to 5. It also changes the third element in the array to a value of 33 (previously 7).
int my_int_array[5] = {3, 2, 5, 7, 3}; int my_var = my_int_array[2]; my_int_array[3] = 33;
Python
Let’s do something similar with Python.
my_initialized_list = [1, "cake", 6.43] my_var = my_initialized_list[1] my_initialized_list[2] = "bacon"
my_var will get the value cake and the second element in the list will get the value bacon (previously 6.43).
Multidimensional Arrays
Adding dimensions can be both easy and more complex, depending on language.
C++/Arduino
Working with multiple dimensions within this family of programming languages is very easy.
float my_table[10][20]; my_table[4][3] = 3.4;
The above code first declares a 10×20 table (two-dimensional array) then sets a specific element in the table (element 4,3) to the value 3.4.
Python
This is sadly a bit more complex in Python, but do not despair!
x = 5 y = 6 my_table = [0]*x for i in range(x): my_table = [0]*y my_table[2][3] = 4
Here we create a list with 5 elements, each having the value of 0. We then use a for loop, as described in part 3 to create a list with 6 elements for each element in the initial list. This results in a 5×6 table with zeros.
Lastly we set a specific element (element (2,3)) to the value of 4, just like in the C++/Arduino example.
List-specific Functions in Python
Python has a lot of useful built-in functions (aka. methods) which you can use to modify your lists. We’re not going to go through them all here, but you can find plenty of info about them around the interwebs (for instance here). One of these is the append() function which we’re going to use in the bottom Python example.
Fibonacci Examples
We’re going to expand on our examples from part 3 where we going to store the values in an array instead of printing them. After that we’ll print out a single element from the array.
C++/Arduino
Serial.begin(115200); int n = 42; long fib_array[n]; int fib_lookup_index = 10; long fib_old = 0; long fib_new = 1; long fib_next = 1; for(int i=0;i<n;i++){ fib_array[i] = fib_old; //insert fib_old at index i fib_old = fib_new; fib_new = fib_next; fib_next = fib_new + fib_old; } Serial.println(fib_array[fib_lookup_index]); //print element 10
Python
In Python we can do this in at least two distinct different ways. The first one is very similar to the C++/Arduino example. We use the Python constant None to represent the absence of a value.
n = 42 fib_array = [None]*n fib_lookup_index = 10 fib_old = 0 fib_new = 1 fib_next = 1 for i in range(0,n): fib_array[i] = fib_old #insert fib_old at index i fib_old = fib_new fib_new = fib_next fib_next = fib_new + fib_old print(fib_array[fib_lookup_index]) #print element 10
Another way to do this is to declare an empty array and use the Python function append() on the array to add elements to the array at the end.
n = 42 fib_array = [] fib_lookup_index = 10 fib_old = 0 fib_new = 1 fib_next = 1 for i in range(0,n): fib_array.append(fib_old) #add a fib_old element at the end of the array fib_old = fib_new fib_new = fib_next fib_next = fib_new + fib_old print(fib_array[fib_lookup_index]) #print element 10
Summary
Using arrays are the most common way to store a set of variables. Python’s lists are the closest you get to the traditional arrays, and they behave a bit differently, not only regarding syntax.
If you’re coding on something without memory protection be careful that you stay within the array. Indexation bugs are quite common when code becomes a bit more complex, so watch your step and remember the zero-indexation.
Continue with part 5: Functions and Scope.