Sometimes you have a noisy signal which you want to low-pass filter to a less noisy signal. The Exponential Moving Average (EMA) filter is a nice and easy filter to implement on your embedded system. Read more about the EMA filter here.
However, sometimes the EMA can be too slow. The trade-off between noise suppression and speed might simply not be good enough. Either the filtered signal doesn’t move fast enough or the signal is too noisy.
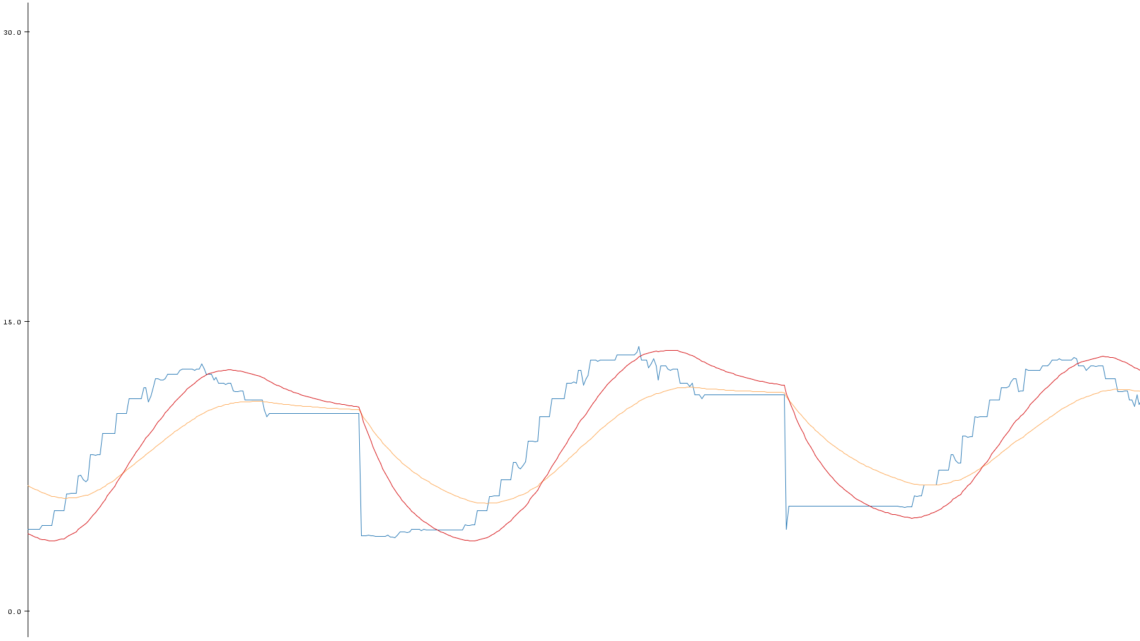
DEMA
…the DEMA is underdamped while the EMA is overdamped.
One solution to this might be the Double Exponential Moving Average (DEMA) filter. At a given α the DEMA has faster response than the EMA while still maintaining the same noise suppression. One potential drawback with the DEMA filter is that you might get an overshoot. In other words the DEMA is underdamped while the EMA is overdamped. Look at this post to find out what we mean by this.
Algorithm
The algorithm is pretty straight forward:
The most tricky thing here is the last term where you take the EMA of the EMA. To achieve this we have to have two global variables instead of one.
Implementation
Here’s a quick implementation of the DEMA filter on Arduino.
float EMA_function(float alpha, int latest, int stored); int sensor_pin = 0; float ema_a = 0.06; int ema_ema = 0; int ema = 0; void setup() { } void loop() { int sensor_value = analogRead(sensor_pin); ema = EMA_function(ema_a, sensor_value, ema); ema_ema = EMA_function(ema_a, ema, ema_ema); int DEMA = 2*ema - ema_ema; delay(1); } float EMA_function(float alpha, int latest, int stored){ return round(alpha*latest) + round((1-alpha)*stored); }
Notice that we use two global variables for the filtering instead of one.
Le Graph
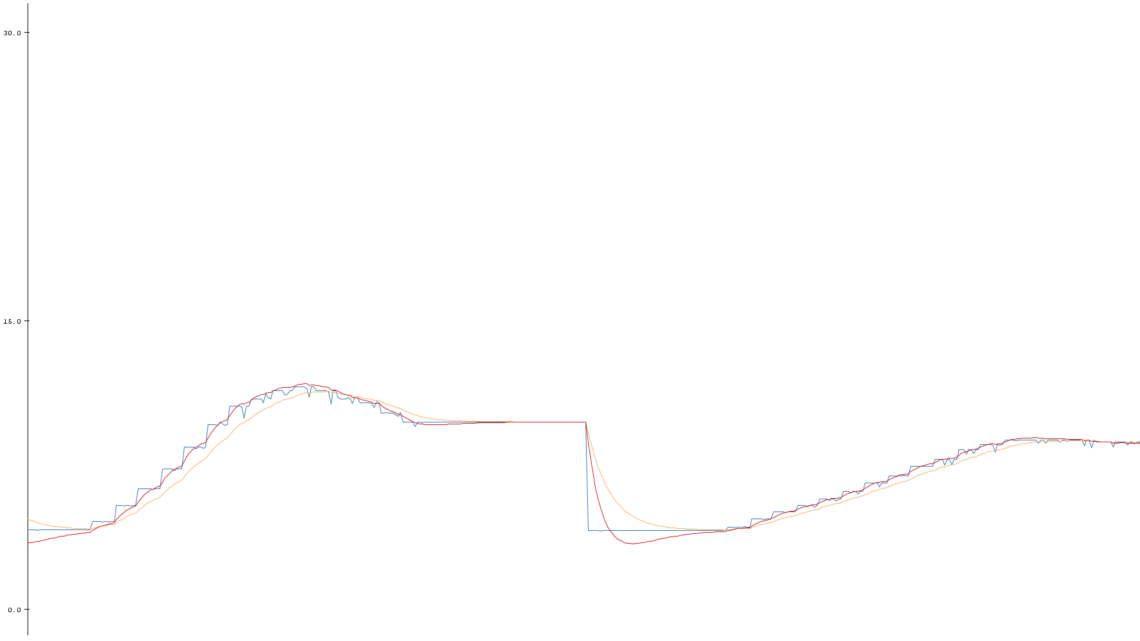
As you can see here, the DEMA manages to follow the original signal better without more noise. Also notice the overshoot at the step response.
The Chapter at the Bottom
The DEMA was tested in the Seesaw project with positive results after writing the final blogpost. This is a scenario where we needed the high speed that the DEMA can provide.
There is also something that’s called Triple Exponential Moving Average (TEMA). This filter uses the same principles as DEMA, but take them even further.